Mastering Laravel Cookies: Setting, Retrieving, and Managing in Your Application
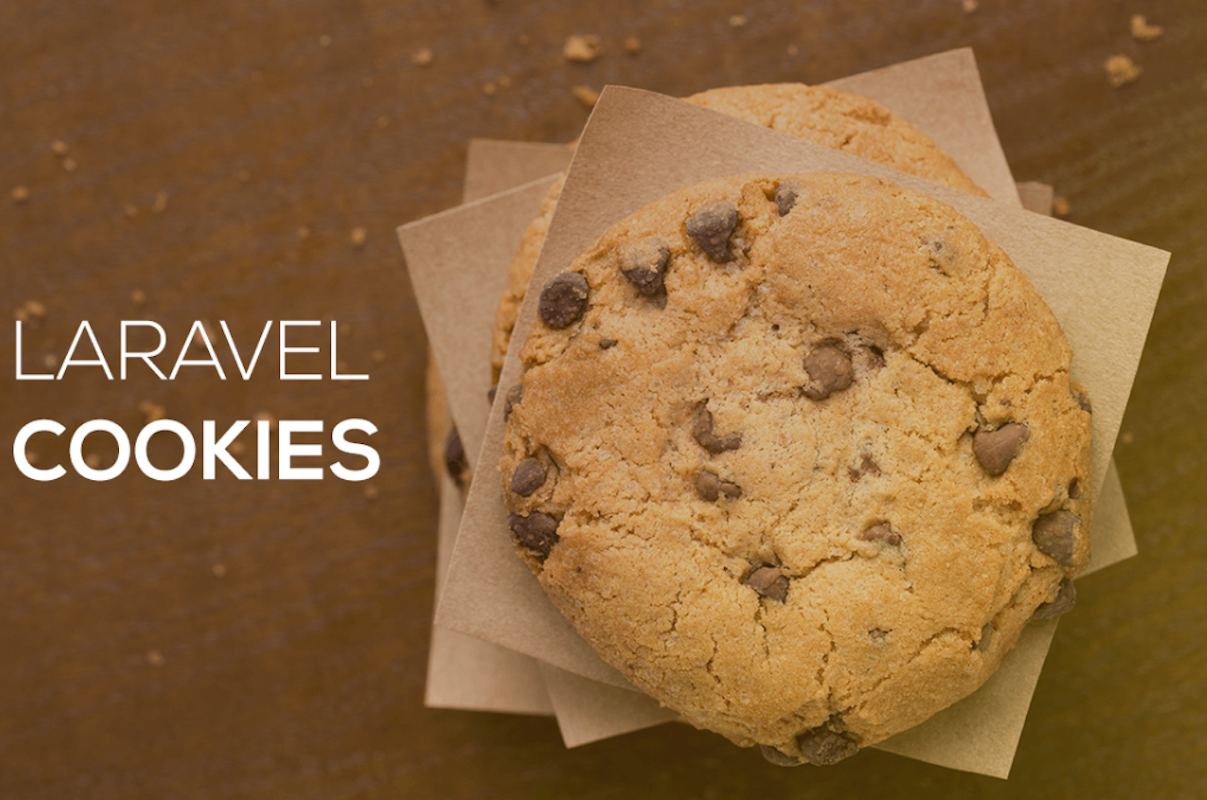
Laravel provides developers with convenient methods for working with cookies, which are small pieces of data stored on the client-side. Cookies are commonly used for various purposes such as session management, user authentication, and tracking user preferences. In this detailed post, we’ll explore how to work with cookies in Laravel, including setting, retrieving, and managing them effectively.
Understanding Laravel Cookies:
Cookies in Laravel are managed through the Illuminate\Http\Response
and Illuminate\Http\Request
classes. Laravel provides a clean and intuitive API for setting and retrieving cookies, making it easy for developers to incorporate cookie functionality into their applications.
Setting Cookies in Laravel:
To set a cookie in Laravel, you can use the cookie()
method available in the Illuminate\Support\Facades\Cookie
facade. This method accepts several parameters, including the name of the cookie, its value, expiration time, path, domain, and secure flag. Here’s an example of how to set a cookie in Laravel:
use Illuminate\Support\Facades\Cookie; Cookie::queue('name', 'value', 60); // Set a cookie named 'name' with a value of 'value' that expires in 60 minutes
You can also set cookies using the response()
helper function, like so:
$response = response('Hello World')->cookie('name', 'value', 60);
Retrieving Cookies in Laravel:
To retrieve a cookie value in Laravel, you can use the Illuminate\Http\Request
instance. Laravel automatically decrypts and decrypts incoming cookies, making them accessible via the cookie()
method. Here’s an example of how to retrieve a cookie value in Laravel:
use Illuminate\Http\Request; $request = Request::capture(); $value = $request->cookie('name');
Deleting Cookies in Laravel:
In Laravel, you can delete a cookie by setting its value to null
and providing an expiration time in the past. This effectively removes the cookie from the client’s browser. Here’s an example of how to delete a cookie in Laravel:
use Illuminate\Support\Facades\Cookie; Cookie::queue(Cookie::forget('name'));
Managing Cookies Across Requests:
By default, cookies set in Laravel are “queued” and sent with the subsequent response. This allows you to set multiple cookies within the same request. However, if you need to immediately set a cookie and access it within the same request, you can use the cookie()
helper function with the response object, as shown earlier.
Security Considerations:
When working with cookies in Laravel, it’s essential to consider security implications, especially when dealing with sensitive information such as authentication tokens or user data. Always ensure that cookies are set with appropriate expiration times, path, and domain restrictions to mitigate security risks.
Working with cookies in Laravel is straightforward and intuitive, thanks to Laravel’s elegant API and convenient helper functions. By understanding how to set, retrieve, and manage cookies effectively, you can enhance the functionality and user experience of your Laravel applications while ensuring security and compliance with best practices.